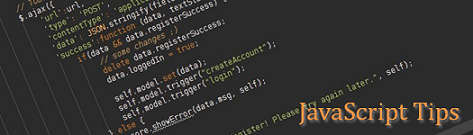
If you are doing lot of JavaScript programming, you might find below list of code snippets very useful. Keep it handy (bookmark it) and save it for future reference. Here are 20 very useful JavaScript tips and tricks for you. Disclaimer: Not all these snippet are written by me. Some of them are collected from other sources on Internet.
1. Converting JavaScript Array to CSV
First one goes like this. You have a javascript array of strings (or numbers) and you want to convert it to comma separated values (csv). We’ll below is the code snippet: Reference: Array to CSV in JavaScript
var fruits = ['apple', 'peaches', 'oranges', 'mangoes'];
var str = fruits.valueOf();
//print str: apple,peaches,oranges,mangoes
Code language: JavaScript (javascript)
The valueOf()
method will convert an array in javascript to a comma separated string. Now what if you want to use pipe (|) as delimeter instead of comma. You can convert a js array into a delimeted string using join()
method. See below:
var fruits = ['apple', 'peaches', 'oranges', 'mangoes'];
var str = fruits.join("|");
//print str: apple|peaches|oranges|mangoes
Code language: JavaScript (javascript)
The join()
method will convert the array into a pipe separated string.
2. Convert CSV to Array in JavaScript
Now what if you want to convert a comma seprated string into a JavaScript array? We’ll there is a method for that too. You can use split()
method to split a string using any token (for instance comma) and create an array. Reference: Array to CSV in JavaScript In below example we split string str
on comma (,) and create a fruitsArray
array.
var str = "apple, peaches, oranges, mangoes";
var fruitsArray = str.split(",");
//print fruitsArray[0]: apple
Code language: JavaScript (javascript)
3. Remove Array element by Index
You have an array. You want to remove a perticular element from array based on its index. We’ll you can do so using splice()
method. This method can add as well as removes element from an array. We will stick to its removal usage. Reference: Remove element by array index
function removeByIndex(arr, index) {
arr.splice(index, 1);
}
test = new Array();
test[0] = 'Apple';
test[1] = 'Ball';
test[2] = 'Cat';
test[3] = 'Dog';
alert("Array before removing elements: "+test);
removeByIndex(test, 2);
alert("Array after removing elements: "+test);
Code language: JavaScript (javascript)
4. Remove Array element by Value
This one is very useful. You have an array and you want to remove an element based on its value (instead of index). Reference: Remove element by array index
function removeByValue(arr, val) {
for(var i=0; i < arr.length; i++) {
if(arr[i] == val) {
arr.splice(i, 1);
break;
}
}
}
var somearray = ["mon", "tue", "wed", "thur"];
removeByValue(somearray, "tue");
//somearray will now have "mon", "wed", "thur"
Code language: JavaScript (javascript)
See how in above code we defined a method removeByValue
that takes serves the purpose. In JavaScript you can define new functions to classes at runtime (although this is discourage) using prototypes
. In below code snippet, we create a new method removeByValue()
within Array
class. So now you can call this method as any other arrays method.
Array.prototype.removeByValue = function(val) {
for(var i=0; i < this.length; i++) {
if(this[i] == val) {
this.splice(i, 1);
break;
}
}
}
//..
var somearray = ["mon", "tue", "wed", "thur"]
somearray.removeByValue("tue");
//somearray will now have "mon", "wed", "thur"
Code language: JavaScript (javascript)
5. Calling JavaScript function from String
Sometime you want to call a Javascript method at runtime whos name you know. Let say there is a method “foo()” which you want to call at runtime. Below is a small JS snippet that helps you calling a method just by its name. Reference: Call Function as String
var strFun = "someFunction"; //Name of the function to be called
var strParam = "this is the parameter"; //Parameters to be passed in function
//Create the function
var fn = window[strFun];
//Call the function
fn(strParam);
Code language: JavaScript (javascript)
6. Generate Random number from 1 to N
Below little snippet helps you in generating random number between 1 to N. Might come handy for your next JS game.
//random number from 1 to N
var random = Math.floor(Math.random() * N + 1);
//random number from 1 to 10
var random = Math.floor(Math.random() * 10 + 1);
//random number from 1 to 100
var random = Math.floor(Math.random() * 100 + 1);
Code language: JavaScript (javascript)
7. Capture browser close button event or exiting the page in JavaScript
This is a common use case. You want to capture the browsers close event so that you can alert user if there is any unsaved data on webpage that should be saved. Below javascript snippet will help you in that. Reference: Capture Browser Close Event
<script language="javascript">
function fnUnloadHandler() {
// Add your code here
alert("Unload event.. Do something to invalidate users session..");
}
</script>
<body onbeforeunload="fnUnloadHandler()">
<!-- Your page content -->
</body>
Code language: HTML, XML (xml)
8. Warn user if Back is pressed
This is same as above. Difference is instead of capturing close event here we capture back button event. So that we know if user is moving awaw from this webpage. Reference: Capture Browser Back Button
window.onbeforeunload = function() {
return "You work will be lost.";
};
Code language: JavaScript (javascript)
9. Check if Form is Dirty
A common usecase. You need to check if user changed anything in an HTML form. Below function formIsDirty
returns a boolean value true or false depending on weather user modified something within the form.
/**
* Determines if a form is dirty by comparing the current value of each element
* with its default value.
*
* @param {Form} form the form to be checked.
* @return {Boolean} <code>true</code> if the form is dirty, <code>false</code>
* otherwise.
*/
function formIsDirty(form) {
for (var i = 0; i < form.elements.length; i++) {
var element = form.elements[i];
var type = element.type;
if (type == "checkbox" || type == "radio") {
if (element.checked != element.defaultChecked) {
return true;
}
}
else if (type == "hidden" || type == "password" ||
type == "text" || type == "textarea") {
if (element.value != element.defaultValue) {
return true;
}
}
else if (type == "select-one" || type == "select-multiple") {
for (var j = 0; j < element.options.length; j++) {
if (element.options[j].selected !=
element.options[j].defaultSelected) {
return true;
}
}
}
}
return false;
}
Code language: JavaScript (javascript)
window.onbeforeunload = function(e) {
e = e || window.event;
if (formIsDirty(document.forms["someForm"])) {
// For IE and Firefox
if (e) {
e.returnValue = "You have unsaved changes.";
}
// For Safari
return "You have unsaved changes.";
}
};
Code language: JavaScript (javascript)
10. Disable Back button using JavaScript
This one is tricky. You want to disable the browsers back button (Dont ask me why!!). Below code snippet will let you do this. The only catch here is that you need to put this code in page where you dont want user to come back. See below reference for more details. Reference: Disable Browsers Back Button
<SCRIPT type="text/javascript">
window.history.forward();
function noBack() { window.history.forward(); }
</SCRIPT>
</HEAD>
<BODY onload="noBack();"
onpageshow="if (event.persisted) noBack();" onunload="">
Code language: HTML, XML (xml)
11. Deleting Multiple Values From Listbox in JavaScript
You have a SELECT box. User can select multiple OPTIONS from this SELECT box and remove them. Below Javascript function selectBoxRemove
let you do this. Just pass ID of SELECT object you want to remove OPTIONS in. Reference: Delete Multiple Options in Select
function selectBoxRemove(sourceID) {
//get the listbox object from id.
var src = document.getElementById(sourceID);
//iterate through each option of the listbox
for(var count= src.options.length-1; count >= 0; count--) {
//if the option is selected, delete the option
if(src.options[count].selected == true) {
try {
src.remove(count, null);
} catch(error) {
src.remove(count);
}
}
}
}
Code language: JavaScript (javascript)
12. Listbox Select All/Deselect All using JavaScript
You can use below JS snippet to select/deselect all the OPTIONS from a SELECT box. Just pass the ID of select box you want to perform this operation on and also a boolean value isSelect
specifying what operation you want to perform. Reference: Select All/None using Javascript
function listboxSelectDeselect(listID, isSelect) {
var listbox = document.getElementById(listID);
for(var count=0; count < listbox.options.length; count++) {
listbox.options[count].selected = isSelect;
}
}
Code language: JavaScript (javascript)
13. Listbox Move selected items Up / Down
This one is useful if you are playing a lot with multi options select box in your application. This function let you move select OPTIONS in a SELECT box to UP or DOWN. See below reference for more details. Reference: Move Up/Down Selected Items in a Listbox
function listbox_move(listID, direction) {
var listbox = document.getElementById(listID);
var selIndex = listbox.selectedIndex;
if(-1 == selIndex) {
alert("Please select an option to move.");
return;
}
var increment = -1;
if(direction == 'up')
increment = -1;
else
increment = 1;
if((selIndex + increment) < 0 ||
(selIndex + increment) > (listbox.options.length-1)) {
return;
}
var selValue = listbox.options[selIndex].value;
var selText = listbox.options[selIndex].text;
listbox.options[selIndex].value = listbox.options[selIndex + increment].value
listbox.options[selIndex].text = listbox.options[selIndex + increment].text
listbox.options[selIndex + increment].value = selValue;
listbox.options[selIndex + increment].text = selText;
listbox.selectedIndex = selIndex + increment;
}
//..
//..
listbox_move('countryList', 'up'); //move up the selected option
listbox_move('countryList', 'down'); //move down the selected option
Code language: JavaScript (javascript)
14. Listbox Move Left/Right Options
This javascript function lets you move selected OPTIONS between two SELECT boxes. Check below reference for details. Reference: Move Options Left/Right between two SELECT boxes
function listbox_moveacross(sourceID, destID) {
var src = document.getElementById(sourceID);
var dest = document.getElementById(destID);
for(var count=0; count > src.options.length; count++) {
if(src.options[count].selected == true) {
var option = src.options[count];
var newOption = document.createElement("option");
newOption.value = option.value;
newOption.text = option.text;
newOption.selected = true;
try {
dest.add(newOption, null); //Standard
src.remove(count, null);
}catch(error) {
dest.add(newOption); // IE only
src.remove(count);
}
count--;
}
}
}
//..
//..
listbox_moveacross('countryList', 'selectedCountryList');
Code language: JavaScript (javascript)
15. Initialize JavaScript Array with series of numbers
Sometime you want to initialize a Javascript array with series of numbers. Below code snippet will let you achieve this. This will initialiaze array numbers
with numbers 1 to 100.
var numbers = [];
for(var i=1; numbers.push(i++)<100;);
//numbers = [0,1,2,3 ... 100]
Code language: JavaScript (javascript)
16. Rounding Numbers to ‘N’ Decimals
This one is quite useful. It will let you round off a number to ‘N’ decimal places. Here in below example we are rounding of a number to 2 decimal places.
var num = 2.443242342;
alert(num.toFixed(2)); // 2.44
Code language: JavaScript (javascript)
Note that we use toFixed()
method here. toFixed(n)
provides n length after the decimal point; whereas toPrecision(x)
provides x total length.
num = 500.2349;
result = num.toPrecision(4); // result will equal 500.2
Code language: JavaScript (javascript)
17. Check if String contains another substring in JavaScript
Below code snippet (courtesy Stackoverflow) let you check if a given string contains another substring. Reference: stackoverflow
if (!Array.prototype.indexOf) {
Array.prototype.indexOf = function(obj, start) {
for (var i = (start || 0), j = this.length; i < j; i++) {
if (this[i] === obj) { return i; }
}
return -1;
}
}
if (!String.prototype.contains) {
String.prototype.contains = function (arg) {
return !!~this.indexOf(arg);
};
}
Code language: JavaScript (javascript)
The code will add two new methods to String, indexOf
and contains
. Once this is done, you can use contains method to check weather a substring is present in a given string.
var hay = "a quick brown fox jumps over lazy dog";
var needle = "jumps";
alert(hay.contains(needle));
Code language: JavaScript (javascript)
18. Remove Duplicates from JavaScript Array
Aha!! You know this one comes quite handy. Just call removeDuplicates method and pass the array, it should remove all duplicate elements from the array.
function removeDuplicates(arr) {
var temp = {};
for (var i = 0; i < arr.length; i++)
temp[arr[i]] = true;
var r = [];
for (var k in temp)
r.push(k);
return r;
}
//Usage
var fruits = ['apple', 'orange', 'peach', 'apple', 'strawberry', 'orange'];
var uniquefruits = removeDuplicates(fruits);
//print uniquefruits ['apple', 'orange', 'peach', 'strawberry'];
Code language: JavaScript (javascript)
19. Trim a String in JavaScript
Below code will add a method trim() to String which can be used to remove spaces from both sides of a string.
if (!String.prototype.trim) {
String.prototype.trim=function() {
return this.replace(/^\s+|\s+$/g, '');
};
}
//usage
var str = " some string ";
str.trim();
//print str = "some string"
Code language: JavaScript (javascript)
20. Redirect webpage in JavaScript
This javascript code should perform http redirect on a given URL.
window.location.href = "//www.viralpatel.net";
Code language: JavaScript (javascript)
21. Encode a URL in JavaScript
Whenever you are making an http request and passing some parameters. You should encode the URL string. Use encodeURIComponent function for encoding a url parameter. Reference: w3scools
var myOtherUrl =
"http://example.com/index.html?url=" + encodeURIComponent(myUrl);
Code language: JavaScript (javascript)
wow..great…this was very useful…but u have missed about functions ,objects …anyhow it was a gud tutorial..
While a “comma-separated” output indeed means the values are output separated by commas it is not a sufficient definition. You must properly handle quoting if the values being output contain (or could possibly contain) a comma as part of their value and for quoted field embedded quotes have to be properly escaped as well.
Using the “pipe” symbol doesn’t cause this need to be ignored but rather significantly reduces the likelihood that you can get away being lazy – since seldom does output contain ” | “.
Converting JavaScript Array to CSV using valueOf() is bullshit. valueOf returns the primitive value of the specified object, which incase of Array is “instance of that array only.”
In your example (demo code), javascript alert is calling toString() method of Array, which is returning string in csv format.
Your `removeByValue` could be a whole simpler by using `indexOf`.
one question how the function of removing duplicate values is working please help as soon as possible.really urgent
wow awesome list of tricks . . . will surely try it
in Number 17 is posible to use case sensitive, I mean to compare also uppercase or lowercase.
string1 = a quick brown fox jumps over
string2 = Fox jumps
Remove Array element by Value
var somearray = [“mon”, “tue”, “wed”, “thur”];
function removeByValue(arr,vals)
{
var indx= arr.IndexOf(vals);
arr.splice(indx, 1);
return arr
}
removeByValue(somearray, “tue”);
For Remove duplicate value from array is easily by
var temp = [];
for (var i = 0; i<arr.length; i++){
if(temp.indexOf(arr[i])==-1){
temp.push(arr[i]);
}
}
return temp;
For remove duplicate value from an array :
var fruits = [‘apple’, ‘orange’, ‘peach’, ‘apple’, ‘strawberry’, ‘orange’];
console.log( […new Set(fruits)] )