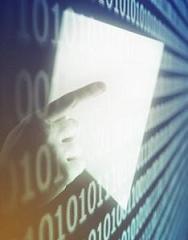
There was a requirement of deleting multiple options from a listbox using JavaScript. User can select multiple items from a Listbox. Once the delete button is pressed, remove all the values that are selected from the combobox. Now the most intuitive solution for this problem would be to iterate through each option from the listbox and delete it if it is selected. So one would write following piece of HTML & JavaScript.
The HTML
Define a simple listbox with id lsbox and two buttons, Delete & Reset.
<table>
<tr>
<td align="center">
<select id="lsbox" name="lsbox" size="10" multiple>
<option value="1">India</option>
<option value="2">United States</option>
<option value="3">China</option>
<option value="4">Italy</option>
<option value="5">Germany</option>
<option value="6">Canada</option>
<option value="7">France</option>
<option value="8">United Kingdom</option>
</select>
</td>
</tr>
<tr>
<td align="center">
<button onclick="listbox_remove('lsbox');">Delete</button>
<button onclick="window.location.reload();">Reset</button>
</td>
</tr>
</table>
Code language: HTML, XML (xml)
Below is the JavaScript code.
The (Incorrect) JavaScript
function listbox_remove(sourceID) {
//get the listbox object from id.
var src = document.getElementById(sourceID);
//iterate through each option of the listbox
for(var count = 0; count < src.options.length; count--) {
//if the option is selected, delete the option
if(src.options[count].selected == true) {
try {
src.remove(count, null);
} catch(error) {
src.remove(count);
}
}
}
}
Code language: JavaScript (javascript)
That’s it right? Wait, this wont work correctly. Each time you delete an option using src.remove()
method, the remaining option’s index will decrease by 1. So if you have selected multiple values to delete, this wont behave as you expected. Check out the below demo:
Demo: The Incorrect Way
In following demo, we used the normal approach (above javascript) to remove the options.
So we know the problem now. Each time the value is deleted, the index of option is decremented. So the ideal approach must be to iterate through the options of listbox in opposite way. Thus we start with the last options and iterate till first.
The Correct JavaScript
Notice the loop highlighted line. We are iterating in opposite direction.
function listbox_remove(sourceID) {
//get the listbox object from id.
var src = document.getElementById(sourceID);
//iterate through each option of the listbox
for(var count= src.options.length-1; count >= 0; count--) {
//if the option is selected, delete the option
if(src.options[count].selected == true) {
try {
src.remove(count, null);
} catch(error) {
src.remove(count);
}
}
}
}
Code language: JavaScript (javascript)
Following is the demo with correct JavaScript.
Demo: The Correct Way
In following demo, we used the correct approach (javascript) to remove the values.
Hope this helps!
Download Source Code
Delete_Multiple_Values_Listbox.zip (1.2 KB)
Update: JQuery Version
It seems that the above code in JavaScript can be replaced with a single JQuery syntax to remove selected items from a listbox. Thanks Ali for the code snippet. Below is the JQuery code for same:
$("#sourceId").find('option:selected').remove();
Code language: JavaScript (javascript)
Nice post Viral..
i this this is crazy way compare with this jquery way
$(“#sourceId”).find(‘option:selected’).remove();
Thanks for the code snippet Ali.. I have added this in above tutorial.
hi…I tried with the Jquery code..it doesn’t work..I believe this is the one instead,
$(“select”).find(“option:selected”).remove();
this works…am not sure…I new to Jquery…let me know …
Thanx for dedication your time in helping others, I highly appreciate !
Just out of curiosity,
On line 10 on the incorrect scripts and line on 11 on the correct script you declared a variable option that isn`t being used at all, any specific reasons as to why you did that !
Ops! thanks for that.. Yes the var option was not required. I have removed it from above code. Thanks again :)
Thanks for the post – I know this is picky since it wasn’t the point of the post, but i think you meant “count++” in the incorrect javascript example (instead of “count–“). Thanks again, this was exactly what i was looking for!
Thanks a lot for sharing your code! I nearly searched all morning for exactly this functionality!
Thanks a lot for sharing your code!
thanks buddy !!!!
Nice Post,
Thanks For sharing it.
Viral how could i transfer the first five options of list box , not by selecting maually from listbox.
Nice buddy!
thanks for your lovely code..cheers
Thanks For sharing it.
very helpful :)
Hello Viral Patel,
I,m trying to add dynamic text,input, drop down on dynamic table row , but when i click to add button the is generated with the widgets but when i select dropdown it shows the the list but when i select any option its shows only first please help how can i do that.
Saved me a lot of time and this made my code much cleaner! Awesome, thanks :)
What is this src.remove(count,null)?
why try catch block?
why not only remove(count)