Spring Boot FreeMarker Hello World Tutorial – Getting started with FreeMarker templates in Spring Boot is super easy. Spring Boot’s auto-configuration (spring starters) speeds up integrating any new tech in any Spring based project. In this tutorial we will learn Spring Boot and FreeMarker integration and create a hello world app.
This Spring Boot app will show a form to capture user input (name, email and date of birth). Show some default values in the table using Freemarker and allow the user to delete the entries from the table. Nothing fancy, the basic stuff.
For this project we will use following technologies:
- Spring Boot 2.1.6
- Java 8 (Can also be compiled with Java 11)
- Maven
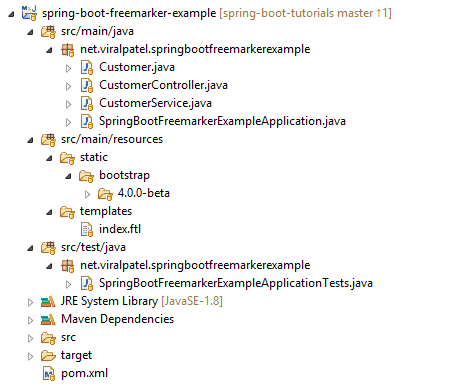
1. Maven dependencies for Spring Boot with FreeMarker
The directory structure is like that of standard Spring Boot project. You could generate this using Spring Initializer (start.spring.io) or just clone this repository. Note that we include the spring-boot-starter-freemarker
to auto configure Freemarker view for Spring Boot web project.
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>net.viralpatel</groupId>
<artifactId>spring-boot-freemarker-example</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>spring-boot-freemarker-example</name>
<description>Spring Boot FreeMarker (FTL) Hello World example</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.6.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<!-- Compile -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Test -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Code language: HTML, XML (xml)
2. Spring Boot Application Class
The Spring Boot application class is the standard one. The Spring Boot Jar project to bootstrap the server and configure default beans. The @SpringBootApplication
is a convenient annotation that wraps @Configuration
, @EnableAutoConfiguration
and @ComponentScan
.
SpringBootFreemarkerExampleApplication.java
package net.viralpatel.springbootfreemarkerexample;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootFreemarkerExampleApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootFreemarkerExampleApplication.class, args);
}
}
Code language: Java (java)
Model and Controller – Spring Boot with FreeMarker
Let’s start with a simple Customer pojo class which contains attributes customerId, customerName, dateOfBirth and email. Also let us add constructor, getter and setter methods.
Customer.java
package net.viralpatel.springbootfreemarkerexample;
import java.time.LocalDate;
import org.springframework.format.annotation.DateTimeFormat;
public class Customer {
private int customerId;
private String customerName;
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE)
private LocalDate dateOfBirth;
private String email;
public Customer() {
super();
}
public Customer(int customerId, String customerName, String email, LocalDate dateOfBirth) {
super();
this.customerId = customerId;
this.customerName = customerName;
this.dateOfBirth = dateOfBirth;
this.email = email;
}
// Getter and setters
}
Code language: Java (java)
Now let us check the CustomerController class. Again, its the standard Spring MVC Controller defined using @Controller
annotation. This class defines methods index()
, add()
and delete()
which are mapped to /, /add and /delete urls respectively. Note that we are using @GetMapping
annotation to map these urls.
Note that in index() method, we return the “index” string. This would render index.ftl freemarker template which we will soon create. Also the @ModelAttribute annotation in index() method binds the modelmap which we can use to pass back the values to freemarker template.
CustomerController.java
package net.viralpatel.springbootfreemarkerexample;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
@Controller
public class CustomerController {
private CustomerService customerService;
public CustomerController(CustomerService customerService) {
this.customerService = customerService;
}
@GetMapping("/")
public String index(@ModelAttribute("model") ModelMap model) {
model.addAttribute("customers", customerService.findAll());
return "index";
}
@PostMapping("/add")
public String add(Customer customer) {
customerService.add(customer);
return "redirect:/";
}
@GetMapping("/delete/{customerId}")
public String delete(@PathVariable int customerId) {
customerService.remove(customerId);
return "redirect:/";
}
}
Code language: Java (java)
Services – Spring Boot with FreeMarker
The CustomerService is again standard Spring @Service
class. We defined a static List of customers. This is just a temporary local data store to keep list of customers. Ideally we would use a database or an in-memory database. However let us keep things simple for now.
CustomerService.java
package net.viralpatel.springbootfreemarkerexample;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.Random;
import org.springframework.stereotype.Service;
@Service
public class CustomerService {
private static List<Customer> customers = new ArrayList<>();
static {
customers.add(new Customer(101, "Steve", "[email protected]", LocalDate.of(1955, 2, 24)));
customers.add(new Customer(201, "Bill", "[email protected]", LocalDate.of(1955, 10, 28)));
customers.add(new Customer(301, "Larry", "[email protected]", LocalDate.of(1973, 8, 21)));
customers.add(new Customer(401, "Sergey", "[email protected]", LocalDate.of(1973, 3, 26)));
}
public List<Customer> findAll() {
return customers;
}
public void add(Customer customer) {
customer.setCustomerId(generateRandomId());
customers.add(customer);
}
private int generateRandomId() {
return new Random().nextInt(1000);
}
public List<Customer> remove(int customerId) {
customers.removeIf(c -> c.getCustomerId() == customerId);
return findAll();
}
public Optional<Customer> find(int customerId) {
return customers.stream().filter(c -> c.getCustomerId() == customerId).findFirst();
}
}
Code language: Java (java)
FreeMarker Template or .ftl file
Finally let us create the freemarker template file to render our view. Create index.ftl file under src/resources folder. This view will render list of customers and a form to add new customer. Note we are using freemarker templates tags <#list>
to loop through customers and render them onto our view.
index.ftl
<!DOCTYPE html>
<html lang="en">
<head>
<title>Spring Boot FreeMarker example - viralpatel.net</title>
<link href="/bootstrap/4.0.0-beta/css/bootstrap.min.css"
rel="stylesheet">
<style>
.container {
margin-top: 80px;
}
.bg-dark {
background-color: #3b8dbd !important;
}
</style>
</head>
<body>
<nav class="navbar navbar-expand-md navbar-dark bg-dark fixed-top">
<a class="navbar-brand" href="https://www.viralpatel.net">Spring Boot
FreeMarker example - viralpatel.net</a>
<button class="navbar-toggler" type="button" data-toggle="collapse"
data-target="#navbarsExampleDefault"
aria-controls="navbarsExampleDefault" aria-expanded="false"
aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
</nav>
<div class="container">
<form class="form-inline" method="post" action="/add">
<input
type="text" class="form-control mb-2 mr-sm-2 mb-sm-0"
id="customerName" name="customerName" placeholder="Customer name" />
<input
type="text" class="form-control mb-2 mr-sm-2 mb-sm-0" id="email"
placeholder="Email" name="email" />
<input type="date"
class="form-control mb-2 mr-sm-2 mb-sm-0" id="dateOfBirth"
placeholder="Birthdate" name="dateOfBirth" />
<button type="submit" class="btn btn-primary">Add</button>
</form>
<br/>
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Customer name</th>
<th>Email</th>
<th>Birthdate</th>
<th></th>
</tr>
</thead>
<tbody>
<#list model["customers"] as customer>
<tr>
<th scope="row">${customer.customerId}</th>
<td>${customer.customerName}</td>
<td>${customer.email}</td>
<td>${customer.dateOfBirth}</td>
<td><a class="btn btn-sm btn-warning" role="button"
href="/delete/${customer.customerId}">Delete</a></td>
</tr>
</#list>
</tbody>
</table>
</div>
</body>
</html>
Code language: HTML, XML (xml)
That’s all folks
Run the Spring Boot Freemarker example project by running Spring Boot Application class as Java class or using maven .\mvnw.cmd spring-boot:run
command in Windows or ./mvnw spring-boot:run
in Mac and Linux. Once the application starts, launch the browser and open: http://localhost:8080/
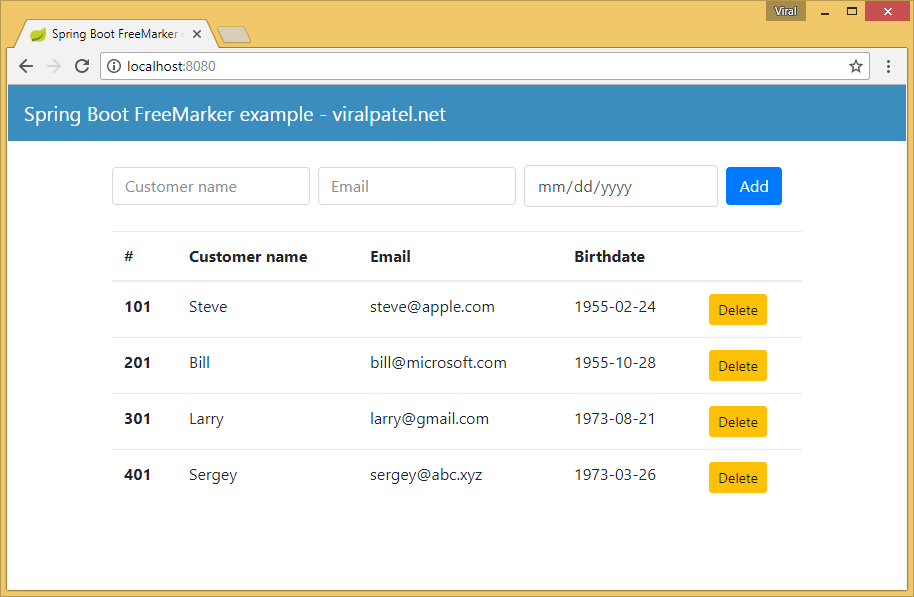
Download Source Code – Spring Boot FreeMarker example
Source code of this Spring Boot FreeMarker Hello World tutorial is available on Github.
Github – spring-boot-freemarker-example
Also read: