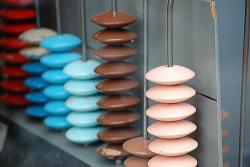
This tutorial guides us on how to pass Array objects from Java to stored procedures in Oracle and also, how to retrieve an array object in Java. All PLSQL arrays can not be called from java. An array needs to be created as TYPE
, at SCHEMA level in the database and then it can be used with ArrayDescriptor in Java, as oracle.sql.ArrayDescriptor
class in Java can not access at package level.
Database Code
First, Create an array, at SCHEMA level. An example is shown below:
CREATE TYPE array_table AS TABLE OF VARCHAR2 (50); -- Array of String
CREATE TYPE array_int AS TABLE OF NUMBER; -- Array of integers
Code language: SQL (Structured Query Language) (sql)
Next, Create a procedure which takes an array as an input parameter and returns an array as its OUT parameter. An example of one such procedure is shown below, which has 2 parameters –
- an array of String as its IN parameter –
p_array
- an array of Integers as OUT parameter –
p_arr_int
CREATE OR REPLACE PROCEDURE SchemaName.proc1 (p_array IN array_table,
len OUT NUMBER,
p_arr_int OUT array_int)
AS
v_count NUMBER;
BEGIN
p_arr_int := NEW array_int ();
p_arr_int.EXTEND (10);
len := p_array.COUNT;
v_count := 0;
FOR i IN 1 .. p_array.COUNT
LOOP
DBMS_OUTPUT.put_line (p_array (i));
p_arr_int (i) := v_count;
v_count := v_count + 1;
END LOOP;
END;
/
Code language: SQL (Structured Query Language) (sql)
After this, Execution permission would be required to execute the procedure created by you:
GRANT EXECUTE ON SchemaNAme.proc1 TO UserName;
Code language: SQL (Structured Query Language) (sql)
Java Code
Create a java class which makes a call to the procedure proc1
, created before. Below is an example which contains the whole flow from creating a connection with the database, to making a call to the stored procedure, passing an array to Oracle procedure, retrieving an array from an Oracle procedure and displaying the result.
import java.math.BigDecimal;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Types;
import oracle.jdbc.OracleCallableStatement;
import oracle.jdbc.internal.OracleTypes;
import oracle.sql.ARRAY;
import oracle.sql.ArrayDescriptor;
public class TestDatabase {
public static void passArray()
{
try{
Class.forName("oracle.jdbc.OracleDriver");
Connection con = DriverManager.getConnection("jdbc:oracle:thin:url ","UserName","Password");;
String array[] = {"one", "two", "three","four"};
ArrayDescriptor des = ArrayDescriptor.createDescriptor("SchemaName.ARRAY_TABLE", con);
ARRAY array_to_pass = new ARRAY(des,con,array);
CallableStatement st = con.prepareCall("call SchemaName.proc1(?,?,?)");
// Passing an array to the procedure -
st.setArray(1, array_to_pass);
st.registerOutParameter(2, Types.INTEGER);
st.registerOutParameter(3,OracleTypes.ARRAY,"SchemaName.ARRAY_INT");
st.execute();
System.out.println("size : "+st.getInt(2));
// Retrieving array from the resultset of the procedure after execution -
ARRAY arr = ((OracleCallableStatement)st).getARRAY(3);
BigDecimal[] recievedArray = (BigDecimal[])(arr.getArray());
for(int i=0;i<recievedArray.length;i++)
System.out.println("element" + i + ":" + recievedArray[i] + "\n");
} catch(Exception e) {
System.out.println(e);
}
}
public static void main(String args[]){
passArray();
}
}
Code language: Java (java)
Brief Explanations:
Class.forName()
– Returns the Class object associated with the class or interface with the given string name.DriverManager.getConnection()
– Attempts to establish a connection to the given database URL.oracle.sql.ArrayDescriptor
– Describes an array classArrayDescriptor.createDescriptor()
– Descriptor factory. Lookup the name in the database, and determine the characteristics of this array.oracle.sql.ARRAY
– An Oracle implementation for generic JDBC Array interface.CallableStatement
– The interface used to execute SQL stored procedures.
References
- http://download.oracle.com/javadb/10.8.1.2/ref/rrefjdbc34565.html
- http://download.oracle.com/javadb/10.4.1.3/ref/rrefjdbc32052.html
- http://download.oracle.com/docs/cd/A97329_03/web.902/q20224/oracle/sql/ArrayDescriptor.html
This is great article
Hi this is sridhar, I executed the above program and stored procedure but I am getting the malformed sql 92 exception I am not getting aware of where the error is whether it is from java side or oracle zide could you please help me thanks for all
Thanks & regards
Sridhar
Hi Viral,
I couldn’t find any reply from you, please help me regarding the above issue.
Hi
I’m Try to execute the above code
i getting error while creating procedure:
CREATE OR REPLACE PROCEDURE SchemaName.proc1 (p_array IN array_table,
len OUT NUMBER,
p_arr_int OUT array_int)
AS
v_count NUMBER;
BEGIN
p_arr_int := NEW array_int ();
p_arr_int.EXTEND (10);
len := p_array.COUNT;
v_count := 0;
FOR i IN 1 .. p_array.COUNT
LOOP
DBMS_OUTPUT.put_line (p_array (i));
p_arr_int (i) := v_count;
v_count := v_count + 1;
END LOOP;
END;
———————————
Error report:
ORA-01435: user does not exist
01435. 00000 – “user does not exist”
*Cause:
*Action:
Try to remove “SchemaName.” from the script, this is only a placeholder.
Very nice article, Vibhati.
However, for this case, I won’t extend the nested table by 10. It should either be extended by p_array.COUNT or each time by 1 inside the loop. It would avoid returning null values.
Hi Thanx,
nice post,
I have tried it but it is showing null or blank. it never show correct data.
Your example use array, can you give an example use ArrayList as input?
I’m afraid you cannot do that. You need to convert arraylist to array and then pass the array to stored procedure.
Hi Joe,
Object –
Array –
Java class.
Employee java class
.i am facing a problem. EmpName is showing blank in DB. but other field is woking fine.
Nice article,,,
Hi,
Is there any way to bind package level associative arrays in java code with hash map.
Please help.
I am trying to do the following.
create or replace
package hr_pkg
as
type charArray is table of varchar2(255) index by varchar2(10);
end;
–Java–
Map test = new HashMap();
test.put(“PLAN”, “2012”);
test.put(“ORDER”, “9999”);
ArrayDescriptor des = ArrayDescriptor.createDescriptor(“hr_pkg.charArray”, con);
ARRAY array_to_pass = new ARRAY(des,con,test);
When i execute this, i recieve
java.sql.SQLException: invalid name pattern: hr_pkg.charArray.
Any work around for this?
Thanks
Thanks for this post, I’m trying to make this work, but I’m not able to succeed :(
here is my example code – modified from your article:
oracle type definition is like this:
oracle proedure:
my problem:
application output looks like this:
database log table contains 8 new rows:
I really don’t understand – when I change String array to int[], (and database type to “table of number”), everything works just fine (log sql table contains A1, A2, A3, A4, B1, B2, B3, B4)… but it is impossible to for me to make it work with string :(
any ideas why is it so?
my oracle driver is ojdbc6-11.2.0.4.0, database version Oracle9i Enterprise Edition Release 9.2.0.8.0 – 64bit Production… every example I have found on the internet claims it works just fine – I really don’t understand
reply to my previous comment: including orai18n.jar should solve the issue – it helped in my case…
Hi Viral,
I have a requirement to pass a null Array to a stored procedure in java using callableStatement.setArray(int, ARRAY). Could you please let me know if it is possible?
– Prasad B
hi. i have a trouble when accessing the array in the stored procedure. i keep getting null values.
Any workaround on this bug. thank you
Hi,
I gone through your article and tried to execute but i couldn’t able to finish sucessfully….
i am facing some problems like….
1. At database level
Permission issue…. which allows me to create synonyms…
2. I am unable to invoke procedure from JAVA program mentioned above …. i am gettting following error :
ORA-01775: looping chain of synonyms
ORA-06512: at “SYS.DBMS_PICKLER”, line 18
ORA-06512: at “SYS.DBMS_PICKLER”, line 58
ORA-06512: at line 1
Can you suggest some solution…. :-)
Hi Viral,
I’m using same as above mentioned in your example but i’m facing issue in Oracle 9i
p_arr_int := NEW array_int (); — ORA-00103
Can you please help with alternate solution to it.
Actually from cursor i’m storing filtered data in PLSQL table which i’m returning is OUT Parameter.
nice post
use orai18n.jar to solve the issue of sending string array to pl/sql procedure
If anyone wants to use the passed in array in the query try this. NOT IN(SELECT * FROM table(array_table));
good article