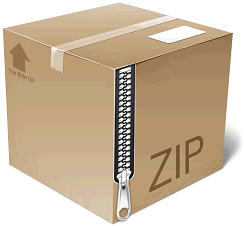
Zip is a very useful file type if I must say most used. It is the most used file format for data compression and archiving. There are number utilities available to create/generate Zip file. Also most of the programming languages comes up with API supporting to generate Zip files. I have written a couple of articles for zipping/unzipping files in Java and PHP.
While browsing internet, I came up to a very interesting website http://jszip.stuartk.co.uk/. This is JavaScript based API to generate Zip files on the fly! It’s very simple to use. All you need to do is to include the jszip javascript file in your HTML document and call its API.
Let us see how to generate a simple ZIP file in JavaScript.
Hello world ZIP in JavaScript
Let us create a helloworld ZIP file which contains two text files, hello1.txt and hello2.txt.
Step 1. Import jszip JavaScript
Include the jszip javascript file in the HTML document where you want to generate ZIP files. You can download the jszip package and include it in HTML or directly include the jszip javascript through git repository.
<script type="text/javascript"
src="https://raw.github.com/Stuk/jszip/master/jszip.js"></script>
Code language: HTML, XML (xml)
Step 2. Generate Hello world ZIP
Following code snippet is HTML file which contains Javascript code to generate ZIP file.
<HTML>
<HEAD>
<script type="text/javascript" src="jszip.js"></script>
</HEAD>
<BODY>
<input type="button" onclick="create_zip()" value="Create Zip"></input>
</BODY>
<SCRIPT>
function create_zip() {
var zip = new JSZip();
zip.add("hello1.txt", "Hello First World\n");
zip.add("hello2.txt", "Hello Second World\n");
content = zip.generate();
location.href="data:application/zip;base64," + content;
}
</SCRIPT>
</HTML>
Code language: HTML, XML (xml)
In above code snippet, we have a button “Create Zip” which calls javascript function create_zip(). This function calls jszip API to generate ZIP file.
Online Demo
Check the online demo.
Filename Problem
If you have tried above demo in Firefox or Safari, you may have noticed the file that is generated has weird name: e.g. b77AewqA7.zip.part. This is because of existing bugs 367231 and 532230. However jszip comes with a unique workaround of this problem by using Downloadify.
zip = new JSZip();
zip.add("Hello.", "hello.txt");
Downloadify.create('downloadify',{
...
data: function(){
return zip.generate();
},
...
dataType: 'base64'
});
Code language: JavaScript (javascript)
Hope this basic demo will help you get going with Client side ZIP file creation in JavaScript.
Hi,
Can u explain me a bit what is the application of above in a website.
This seems a really nice project. Does it works on any Android browsers?
wtf with that downloadify link?
This solution doesn’t work with internet explorer. It works for Chrome and Safari. It’s not useful if you have to tell people to stop using Internet Explorer.
@Jason: It will work with IE10 with a minor modification.
when using this code in my application it not create zip file but create file with extension part ?????????
Dear All,
In order to understand what is going wrong in IE9, I tried debugging. Assigning the result of zip.generate() to the variable content generated an error: SCRIPT438: Object doesn’t support property or method. However, when I replace the first line of html with this:
then the exception does not fire. See also:
http://msdn.microsoft.com/en-us/library/cc288325(v=vs.85).aspx
Instead my browser is redirected to an URL starting with:
data:application/zip;base64,UEsDBAoAAAAAA…
If we can make sure the URL is recognised as a download, we can fix this problem!
Kind regards
Hi, Thanks for your reply. Please let me know if this is working for IE version below 9. If yes, can you send me the piece of code([email protected]).
Unfortunately, 2 lines of those I typed were not displayed. Another try:
Hi viral,can u explain how to send SMS using java.
Hey Hi Viral Patel, Very nice Tutorial dear……..
Thank You so much…..
Hi,
This works fine!
However, how would I get it to write an image to the .zip file.
E.g I have Images on a page and I want to save them to a .zip
Hi there, when doing zip.add() I get the error: Object # has no method ‘add’
It’s probably best to download the javascript and host it for yourself. Github isn’t meant to be hotlinked to like that, if the author changes the filename, repository name, their username or removes the repository then you will end up with a 404 error in your page.
Hi viral,
Thanx for posting this, i’ve tried researching web for 2 days and finally found this article, its very useful for me. i just wanted to know is there any function in your JS where i can secure zip file with password and unlock it again as i wanted?
Help appreciated.
good one.. JS is free open source right?
it’s really a useful tool.i care about how to use it exactly, so i wish you could provide more demo.
Can you please tell me if I want to zip my local system file how could I do it???
This code is not working with safari windows. The name and the type of the file is unknown.
Can you please let me know how to zip a file if selected it with field and how can I upload zip file to server. If you can help me with a code it will be great help for me.
How can i zip a pdf file from local directory. because in your example there is only text file and whose content is written there.
please suggest.
Thanks
Hi.
I cannot add image to zip
I use example: zip.folder(“images”).add() but not work
thanks
Hi guy, my proxy block the
How can I do ? Thanks
Hi guy, my proxy block the https://raw.github.com/Stuk/jszip/master/jszip.js How can I do ? thanks
Hi How to upload a zip file directly to another server directly using web api (eg.Google Drive, etc) instead to download to local file?
how can I make the zip file itself
I want to Name the zip file itself
Very nice article. ….I wondered to find this script.
How can I create zip file from multiple files on server/mysql (/uploads) directory and download files by specific user id who uploaded it