Let us understand how One-to-one relationship is implemented in Hibernate using Annotations. For this we
will use our previous article One-to-one mapping in Hibernate using XML mapping and enhance it to support Annotations.
Tools and Technologies used in this article:
- Java JDK 1.5 above
- MySQL 5 above
- Eclipse 3.2 above
- Hibernate 3 above
- Maven 3 above
1. Database with One-to-one relationship tables
We will use the same tables we created in our previous article for this example. Following is the SQL code:
/* EMPLOYEE table */
CREATE TABLE `employee` (
`employee_id` BIGINT(10) NOT NULL AUTO_INCREMENT,
`firstname` VARCHAR(50) NULL DEFAULT NULL,
`lastname` VARCHAR(50) NULL DEFAULT NULL,
`birth_date` DATE NOT NULL,
`cell_phone` VARCHAR(15) NOT NULL,
PRIMARY KEY (`employee_id`)
)
COLLATE='latin1_swedish_ci'
ENGINE=InnoDB
ROW_FORMAT=DEFAULT
AUTO_INCREMENT=216
/* EMPLOYEEDETAIL table */
CREATE TABLE `employeedetail` (
`employee_id` BIGINT(20) NOT NULL AUTO_INCREMENT,
`street` VARCHAR(50) NULL DEFAULT NULL,
`city` VARCHAR(50) NULL DEFAULT NULL,
`state` VARCHAR(50) NULL DEFAULT NULL,
`country` VARCHAR(50) NULL DEFAULT NULL,
PRIMARY KEY (`employee_id`),
CONSTRAINT `FKEMPL` FOREIGN KEY (`employee_id`) REFERENCES `employee` (`employee_id`)
)
COLLATE='latin1_swedish_ci'
ENGINE=InnoDB
ROW_FORMAT=DEFAULT
AUTO_INCREMENT=216
Code language: SQL (Structured Query Language) (sql)
So we have created two tables “EMPLOYEE” and “EMPLOYEEDETAILS” which have One-to-one relational mapping. These two tables are mapped by primary key Employee_ID.
2. Model class to Entity class
We had define two model classes Employee.java and EmployeeDetail.java in our previous example. These classes will be converted to Entity classes and we will add Annotations to it.
File: /src/main/java/net/viralpatel/hibernate/Employee.java
package net.viralpatel.hibernate;
import java.sql.Date;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="EMPLOYEE")
public class Employee {
@Id
@GeneratedValue
@Column(name="employee_id")
private Long employeeId;
@Column(name="firstname")
private String firstname;
@Column(name="lastname")
private String lastname;
@Column(name="birth_date")
private Date birthDate;
@Column(name="cell_phone")
private String cellphone;
@OneToOne(mappedBy="employee", cascade=CascadeType.ALL)
private EmployeeDetail employeeDetail;
public Employee() {
}
public Employee(String firstname, String lastname, Date birthdate, String phone) {
this.firstname = firstname;
this.lastname = lastname;
this.birthDate = birthdate;
this.cellphone = phone;
}
// Getter and Setter methods
}
Code language: Java (java)
File: /src/main/java/net/viralpatel/hibernate/EmployeeDetail.java
package net.viralpatel.hibernate;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.PrimaryKeyJoinColumn;
import javax.persistence.Table;
import org.hibernate.annotations.GenericGenerator;
import org.hibernate.annotations.Parameter;
@Entity
@Table(name="EMPLOYEEDETAIL")
public class EmployeeDetail {
@Id
@Column(name="employee_id", unique=true, nullable=false)
@GeneratedValue(generator="gen")
@GenericGenerator(name="gen", strategy="foreign", parameters=@Parameter(name="property", value="employee"))
private Long employeeId;
@Column(name="street")
private String street;
@Column(name="city")
private String city;
@Column(name="state")
private String state;
@Column(name="country")
private String country;
@OneToOne
@PrimaryKeyJoinColumn
private Employee employee;
public EmployeeDetail() {
}
public EmployeeDetail(String street, String city, String state, String country) {
this.street = street;
this.city = city;
this.state = state;
this.country = country;
}
// Getter and Setter methods
}
Code language: Java (java)
Note that in EmployeeDetail
class we have used @GenericGenerator
to specify primary key. This will ensure that the primary key from Employee table is used instead of generating a new one.
3. Remove Hibernate Mapping (hbm) Files
Delete the hibernate mapping files Employee.hbm.xml
and EmployeeDetail.hbm.xml
as we do not need them anymore. The mapping is now defined in Java class as Annotation.
4. Update Hibernate Configuration
Edit Hibernate configuration file (hibernate.cfg.xml) and add mappings for Employee and EmployeeDetail classes. Following is the final hibernate.cfg.xml file:
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/tutorial</property>
<property name="connection.username">root</property>
<property name="connection.password"></property>
<property name="connection.pool_size">1</property>
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="current_session_context_class">thread</property>
<property name="cache.provider_class">org.hibernate.cache.NoCacheProvider</property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">validate</property>
<mapping class="net.viralpatel.hibernate.EmployeeDetail"/>
<mapping class="net.viralpatel.hibernate.Employee"/>
</session-factory>
</hibernate-configuration>
Code language: HTML, XML (xml)
5. Update Hibernate Dependency in Maven
Update the Maven pom.xml and define following Hibernate dependency into it:
<?xml version="1.0" encoding="UTF-8"?><project>
<modelVersion>4.0.0</modelVersion>
<groupId>HibernateCache</groupId>
<artifactId>HibernateCache</artifactId>
<version>0.0.1-SNAPSHOT</version>
<description></description>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>ejb3-persistence</artifactId>
<version>1.0.1.GA</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-annotations</artifactId>
<version>3.3.1.GA</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.10</version>
</dependency>
</dependencies>
</project>
Code language: HTML, XML (xml)
6. Update Hibernate Utility class
In previous example, the Hibernate Utility class uses Hibernate’s org.hibernate.cfg.Configuration
class to generate SessionFactory. For this example we will replace this class with org.hibernate.cfg.AnnotationConfiguration
class. Following is the code for HibernateUtil.java
class.
File: /src/main/java/net/viralpatel/hibernate/HibernateUtil.java
package net.viralpatel.hibernate;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.AnnotationConfiguration;
public class HibernateUtil {
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
try {
// Create the SessionFactory from hibernate.cfg.xml
return new AnnotationConfiguration().configure()
.buildSessionFactory();
} catch (Throwable ex) {
System.err.println("Initial SessionFactory creation failed." + ex);
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
Code language: Java (java)
7. Main class to test One-to-one mapping
Execute following Main class to test One-to-one relational mapping using Annotation.
File: /src/main/java/net/viralpatel/hibernate/Main.java
package net.viralpatel.hibernate;
import java.sql.Date;
import java.util.List;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
public class Main {
@SuppressWarnings("unchecked")
public static void main(String[] args) {
System.out.println("Hibernate One-To-One example (Annotation)");
SessionFactory sf = HibernateUtil.getSessionFactory();
Session session = sf.openSession();
session.beginTransaction();
EmployeeDetail employeeDetail = new EmployeeDetail("10th Street", "LA", "San Francisco", "U.S.");
Employee employee = new Employee("Nina", "Mayers", new Date(121212),
"114-857-965");
employee.setEmployeeDetail(employeeDetail);
employeeDetail.setEmployee(employee);
session.save(employee);
List<Employee> employees = session.createQuery("from Employee").list();
for (Employee employee1 : employees) {
System.out.println(employee1.getFirstname() + " , "
+ employee1.getLastname() + ", "
+ employee1.getEmployeeDetail().getState());
}
session.getTransaction().commit();
session.close();
}
}
Code language: Java (java)
8. Review Final Project Structure
The final project structure should looks like following:
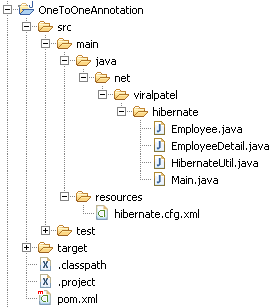
Execute Main Class
Execute the Main class and find the below output.
Output:
Hibernate One-To-One example (Annotation)
Hibernate: insert into EMPLOYEE (birth_date, cell_phone, firstname, lastname) values (?, ?, ?, ?)
Hibernate: insert into EMPLOYEEDETAIL (city, country, state, street, employee_id) values (?, ?, ?, ?, ?)
Hibernate: select employee0_.employee_id as employee1_1_, employee0_.birth_date as birth2_1_, employee0_.cell_phone as cell3_1_, employee0_.firstname as firstname1_, employee0_.lastname as lastname1_ from EMPLOYEE employee0_
Nina , Mayers, San Francisco
Code language: SQL (Structured Query Language) (sql)
Download Source Code
Hibernate-One-to-one-tutorial-Annotations.zip (8 kb)
hi this is guptha
this tutorial help me a lot
i wish to know how configure Hibernate 3 above Maven 3 above in eclipse
please help me
thank you
This is a very, very good tutorial. One of the difficulty is which strategy to use and the relationship, because that all depends on how deep is your existing table model.
I been using bottom up approach but many time don’t know how to map.
You did an excellent job because it is short and easy to read. You have the database script, model diagram. All is all in one very clear from end to end, programmaticly and visually.
Excellent tutorial, thanks a lot!
simple, straightforward, easy to understand.
thanks a lots
Your tutorial is really good. But it would be helpful if you mention which all jars (versions) to be included in the build path
Very Nice tutorial..
in the date place instead of the whole date ( date with time) i want only date .
i have tried like this…
can u pls suggest me?
I would suggest to leave hibernate mapping like this only. Fetch whole Date with Time. In your view format the date and print only date part.
i am using jqgrid.
so can u tell me how to format in gridcolumn.
how to run this example in eclipse …
Run on Server or Run Java Application which of them…
I want to know about the objects that is carried by the page to action and then controller…
thanks for your sample .. that is good ..
Thanks anyway ,,,
log4j:WARN No appenders could be found for logger (com.opensymphony.xwork2.config.providers.XmlConfigurationProvider).
log4j:WARN Please initialize the log4j system properly.
Initial SessionFactory creation failed.java.lang.NullPointerException
Aug 15, 2012 2:23:02 PM org.apache.catalina.core.StandardWrapperValve invoke
SEVERE: Servlet.service() for servlet default threw exception
java.lang.NullPointerException
at org.hibernate.cfg.OneToOneSecondPass.doSecondPass(OneToOneSecondPass.java:135)
at org.hibernate.cfg.Configuration.secondPassCompile(Configuration.java:1163)
at org.hibernate.cfg.AnnotationConfiguration.secondPassCompile(AnnotationConfiguration.java:296)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:1319)
at net.viralpatel.contact.util.HibernateUtil.buildSessionFactory(HibernateUtil.java:14)
at net.viralpatel.contact.util.HibernateUtil.(HibernateUtil.java:8)
at net.viralpatel.contact.view.ContactAction.(ContactAction.java:22)
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(Unknown Source)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(Unknown Source)
at java.lang.reflect.Constructor.newInstance(Unknown Source)
at java.lang.Class.newInstance0(Unknown Source)
at java.lang.Class.newInstance(Unknown Source)
at com.opensymphony.xwork2.ObjectFactory.buildBean(ObjectFactory.java:119)
at com.opensymphony.xwork2.ObjectFactory.buildBean(ObjectFactory.java:150)
at com.opensymphony.xwork2.ObjectFactory.buildBean(ObjectFactory.java:139)
at com.opensymphony.xwork2.ObjectFactory.buildAction(ObjectFactory.java:109)
at com.opensymphony.xwork2.DefaultActionInvocation.createAction(DefaultActionInvocation.java:288)
at com.opensymphony.xwork2.DefaultActionInvocation.init(DefaultActionInvocation.java:388)
at com.opensymphony.xwork2.DefaultActionProxy.prepare(DefaultActionProxy.java:187)
at org.apache.struts2.impl.StrutsActionProxy.prepare(StrutsActionProxy.java:61)
at org.apache.struts2.impl.StrutsActionProxyFactory.createActionProxy(StrutsActionProxyFactory.java:39)
at com.opensymphony.xwork2.DefaultActionProxyFactory.createActionProxy(DefaultActionProxyFactory.java:47)
at org.apache.struts2.dispatcher.Dispatcher.serviceAction(Dispatcher.java:478)
at org.apache.struts2.dispatcher.FilterDispatcher.doFilter(FilterDispatcher.java:395)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:235)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:206)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:233)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:191)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:127)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:102)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:109)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:298)
at org.apache.coyote.http11.Http11Processor.process(Http11Processor.java:859)
at org.apache.coyote.http11.Http11Protocol$Http11ConnectionHandler.process(Http11Protocol.java:588)
at org.apache.tomcat.util.net.JIoEndpoint$Worker.run(JIoEndpoint.java:489)
at java.lang.Thread.run(Unknown Source)
this is my error
pls suggest me
thanks
I want to know about the view.. How many jsp pages will be there??
i am executed one to one relation ship using mapping files successfully executed,but by using annotations i have some problems because my annotations are not supported to my project(i am using myeclise8.6 given default annotations.so please help me where the corresponding annotaions are available.plesse send me link
Hi Viral,
Thanks again for nice tutorial. I want to retrieve all employees from a particular city (Say Mumbai). Can you please let me know what changes are required. I have tried but no luck.
– Shirish
Hi,
Very Nice tutorial..
Thanmk You
Hi Viral,
I’ve tried running your example but making changes to access Oracle instead of MySQL.
In Employee.java:
I’ve created a sequence and a trigger in Oracle and changed also the necessary in the hibernate.cfg.xml file.
Running the application the sequence is accesed but although it is inside a transaction it seems like the value of the sequence retrieved is not available for the employee_detail insert.
Can you help me?
What I get is
Did u try @PrimaryKeyJoinColumn on the detail table? Looks like Hibernate does not know where to refer.
it clearly says that “integrity constraint (HIBERNATE_OWN.EMPLOYEEDETAIL_EMPLOYEE_FK1) violated – parent key not found”
you should create a matching record…only then fetch would work…if the same example is been carried out then you would face no issues..
check whether the constraint is been added in the oracle table employeeDetails
Hi Viral,
In a working application i found configuration like below. They didnt mention column name. How it will work? Help me to understand.
@Column(length = 30)
private String country;
Jolly good tutorial!
Great tutorial. Helps us very much. Thank you for the effort.
Regards,
Premkumar. A
nice tutorial Viral !
will this code work for hibernate for all update() scenarios ? I am using hibernate 3.6
and checking update:
1. I create Employee without EmployeeDetails- success Eemployee is created without EmployeeDetails)
2. when i update Employee (without EmployeeDetails) – success (Employee is updated)
3. when i update employee with EmployeeDetails – FAILED
I am having a similar mapping in my application and failing on #3 requirement. I want to create address during update, when it is not there in address table.
I am calling first getSession().get(persistentClass, id); and then making a call to getSession().update(object). do i have to chage the update call to getSession().merge(object)?
Please respond if the same thing works in your code? if not, then with what changes it should work. Am I missing something in my code?? need to look..
thanks for your time and brilliant efforts on such a nice tutorial !!
Regards,
Akhilesh
missed one information: I am using postgres and not mysql
These articles are awesome, i learned a lot with them. Thank you so much. Maybe in the future i can contribute with some interesting articles too, to help the community,
Are they Bidirectional mappings or Unidirectional mapping ?
I hope it is bidirectional mapping.
Where can i find information about the unidirectional mapping?
Thanks,
Sandy
This article was a life-saver for me when I was stuck in a shared primary key bind.
Nice article very succinct and easy to follow example.
nice tutorial to start with.
nice tutorial
Exception in thread “main” java.lang.NullPointerException
at org.hibernate.tuple.entity.AbstractEntityTuplizer.getPropertyValue(AbstractEntityTuplizer.java:645)
at org.hibernate.persister.entity.AbstractEntityPersister.getPropertyValue(AbstractEntityPersister.java:4481)
at org.hibernate.id.ForeignGenerator.generate(ForeignGenerator.java:99)
at org.hibernate.event.internal.AbstractSaveEventListener.saveWithGeneratedId(AbstractSaveEventListener.java:118)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.saveWithGeneratedOrRequestedId(DefaultSaveOrUpdateEventListener.java:204)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.entityIsTransient(DefaultSaveOrUpdateEventListener.java:189)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.performSaveOrUpdate(DefaultSaveOrUpdateEventListener.java:114)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.onSaveOrUpdate(DefaultSaveOrUpdateEventListener.java:90)
at org.hibernate.internal.SessionImpl.fireSaveOrUpdate(SessionImpl.java:727)
at org.hibernate.internal.SessionImpl.saveOrUpdate(SessionImpl.java:719)
at org.hibernate.engine.spi.CascadingAction$5.cascade(CascadingAction.java:258)
at org.hibernate.engine.internal.Cascade.cascadeToOne(Cascade.java:383)
at org.hibernate.engine.internal.Cascade.cascadeAssociation(Cascade.java:326)
at org.hibernate.engine.internal.Cascade.cascadeProperty(Cascade.java:208)
at org.hibernate.engine.internal.Cascade.cascade(Cascade.java:165)
at org.hibernate.event.internal.AbstractSaveEventListener.cascadeAfterSave(AbstractSaveEventListener.java:448)
at org.hibernate.event.internal.AbstractSaveEventListener.performSaveOrReplicate(AbstractSaveEventListener.java:293)
at org.hibernate.event.internal.AbstractSaveEventListener.performSave(AbstractSaveEventListener.java:193)
at org.hibernate.event.internal.AbstractSaveEventListener.saveWithGeneratedId(AbstractSaveEventListener.java:126)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.saveWithGeneratedOrRequestedId(DefaultSaveOrUpdateEventListener.java:204)
at org.hibernate.event.internal.DefaultSaveEventListener.saveWithGeneratedOrRequestedId(DefaultSaveEventListener.java:55)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.entityIsTransient(DefaultSaveOrUpdateEventListener.java:189)
at org.hibernate.event.internal.DefaultSaveEventListener.performSaveOrUpdate(DefaultSaveEventListener.java:49)
at org.hibernate.event.internal.DefaultSaveOrUpdateEventListener.onSaveOrUpdate(DefaultSaveOrUpdateEventListener.java:90)
at org.hibernate.internal.SessionImpl.fireSave(SessionImpl.java:756)
at org.hibernate.internal.SessionImpl.save(SessionImpl.java:748)
at org.hibernate.internal.SessionImpl.save(SessionImpl.java:744)
at Hibernate.HibernateSampleCode.EmployeeDetailsServices.main(EmployeeDetailsServices.java:46)
Hi i m getting null pointer exception please help me
I get stuck at creating database. I dont understand how you can make a FK between a BIGINT(10) and the other is BIGINT(20), and they are all PRIMARY KEY at first. SQL doesnt let me excute the SQL code block either.
thank you very much.
thanx viral
In your sample, I replace the first code by the second one. Project is still right. But I dont know how difference between them. Can you tell me about that?
the first (it’s yours):
the second:
The second is more simple than former one.
Hi,
I did not execute DDL explicitly.
If I use annotation instead of xml file, table is not creating automatically.
It throwing exception ” java.sql.BatchUpdateException: ORA-00942: table or view does not exist”
I am unable to find solution.Please help me.
Hi Viral,
I have a query regarding these two lines
employee.setEmployeeDetail(employeeDetail);
employeeDetail.setEmployee(employee);
I understand the meaning n necessity of the 1st line of code but don’t understand the same for the second line. Would u please explain why is the second line required? Sorry i am new to these concepts.
Will hibernate generate a mapping file internally if annotations are used.
nice tutorial write one book with the head first
I want one to one mapping of hibernate using spring mvc please any body help me …..
I want one to one mapping of hibernate using spring mvc using Single Controller class in Maven please any body help me …..
getting error like this
Initial SessionFactory creation failed.org.hibernate.AnnotationException: Unknown mappedBy in: com.viralpatel.employee.Employee.employeeDetail, referenced property unknown: com.viralpatel.employee.EmployeeDetail.com.viralpatel.employee.Employee
Exception in thread “main” java.lang.ExceptionInInitializerError
at com.viralpatel.employee.HibernateUtil.buildSessionFactory(HibernateUtil.java:23)
at com.viralpatel.employee.HibernateUtil.(HibernateUtil.java:13)
at com.viralpatel.employee.EmployeeMain.addEmployeeDetails(EmployeeMain.java:20)
at com.viralpatel.employee.EmployeeMain.main(EmployeeMain.java:46)
Caused by: org.hibernate.AnnotationException: Unknown mappedBy in: com.viralpatel.employee.Employee.employeeDetail, referenced property unknown: com.viralpatel.employee.EmployeeDetail.com.viralpatel.employee.Employee
at org.hibernate.cfg.OneToOneSecondPass.doSecondPass(OneToOneSecondPass.java:152)
at org.hibernate.cfg.Configuration.secondPassCompile(Configuration.java:1221)
at org.hibernate.cfg.AnnotationConfiguration.secondPassCompile(AnnotationConfiguration.java:383)
at org.hibernate.cfg.Configuration.buildSessionFactory(Configuration.java:1377)
at org.hibernate.cfg.AnnotationConfiguration.buildSessionFactory(AnnotationConfiguration.java:954)
at com.viralpatel.employee.HibernateUtil.buildSessionFactory(HibernateUtil.java:20)
… 3 more
can you give an example for , storing list as string in db and retreiveing string as list from db, using hibbernate annotations mapping class, dao class and pojo class
Hi thanks for the tutorial. Its is good.
I am facing a problem when I want to insert or update information for an employee without set employee detail information or I should leave it null.
In my database its value is set as null. Not require field for fk concept.
Please can you help me if possible.
Thanks
thanks viralpatel
Hibernate One-To-One example (Annotation)
Initial SessionFactory creation failed.java.lang.NoClassDefFoundError: org/dom4j/DocumentException
Exception in thread “main” java.lang.ExceptionInInitializerError
at one.Utility.buildSessionFactory(Utility.java:18)
at one.Utility.(Utility.java:8)
at one.Main.main(Main.java:18)
Caused by: java.lang.NoClassDefFoundError: org/dom4j/DocumentException
at one.Utility.buildSessionFactory(Utility.java:13)
… 2 more
Caused by: java.lang.ClassNotFoundException: org.dom4j.DocumentException
at java.net.URLClassLoader$1.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClassInternal(Unknown Source)
… 3 more
Thanks viralpatel! Excellent material!
Thanks for the info Viralpatel! It’s a great work.
Gratz
I have two classes i.e Student and Teacher and one Address table . I need to make one to one mapping of student and teacher class with the address table . For teacher class its working fine but for student class Its throwing the error that the instace of Student is altered from 13 to 13 , where 13 is the number from the sequence . Please assist me here . Thanks in advance .
Can you please explain one to one relation with Oracle database, how to use generator class in case of oracle
Really good one
HI
I tried this source code with set hbm2ddl.auto = update to auto create my tables.
Tables are created successfully and data is also inserted in proper way.
but foreign key constraints are not set.
Can you help out on how to auto create tables with foreign key constraints are set properly.
Thanks in advance.
Thanks for the Article.