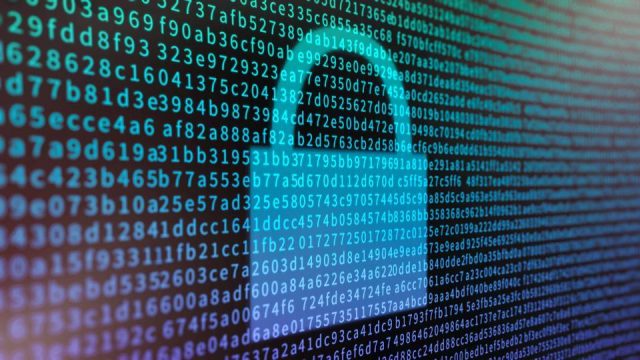
Java URL Encoder/Decoder Example – In this tutorial we will see how to URL encode/decode attributes in Java.
Overview
URL encoding, is a mechanism for encoding information in a Uniform Resource Identifier (URI) under certain circumstances.
When we submit an HTML form using GET or POST request, the data/fields in the form are encoded using application/x-www-form-urlencoded type. There is an optional attribute on <form>
element called enctype
. Its default value is application/x-www-form-urlencoded
. This specification defines how the values are encoded (for e.g. replacing space with +) and decoded on server.
When HTML form data is sent in an HTTP GET request, it is included in the query component of the request URI. When sent in an HTTP POST request, the data is placed in the request body, and application/x-www-form-urlencoded
is included in the message’s Content-Type header.
Encode URL in Java
To encode the query parameters or attributes, Java provides a utility class URLEncoder
with a couple of encode()
methods.
The URLEncoder.encode()
method escapes all characters except:
Code language: Shell Session (shell)A-Z a-z 0-9 - _ . ! ~ * ' ( )
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
//..
URLEncoder.encode(plainText, StandardCharsets.UTF_8.toString());
Code language: Java (java)
In following example, we encode a few plain text strings using URLEncoder.encode.
URLEncoderTest.java
package net.viralpatel;
import org.junit.Test;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
public class URLEncoderTest {
private static final Logger LOGGER = LoggerFactory.getLogger(URLEncoderTest.class);
public static void encode(String plain) throws UnsupportedEncodingException {
String encoded = URLEncoder.encode(plain, StandardCharsets.UTF_8.toString());
LOGGER.info("Plain text: {}, Encoded text: {}", plain, encoded);
}
@Test
public void encodeTests() throws UnsupportedEncodingException {
encode("[email protected]");
encode("sample text");
encode("+1653-124-23");
}
}
Code language: Java (java)
Run the above test in your favourite IDE and check the output. The plain text is encoded using Java URL Encoder.
Output:
Code language: Shell Session (shell)Plain text: [email protected], Encoded text: john%2Bdoe%40example.com Plain text: sample text, Encoded text: sample+text Plain text: +1653-124-23, Encoded text: %2B1653-124-23
Decode URL in Java
Java also provides utility class URLDecoder to decode the value. Usually on server side when we receive the form attributes, using URLDecoder we can decode them to original values.
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.nio.charset.StandardCharsets;
//..
URLDecoder.decode(encodedText, StandardCharsets.UTF_8.toString());
Code language: Java (java)
URLDecoderTest.java
package net.viralpatel;
import org.junit.Test;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.nio.charset.StandardCharsets;
public class URLDecoderTest {
private static final Logger LOGGER = LoggerFactory.getLogger(URLEncoderTest.class);
public static void decode(String encoded) throws UnsupportedEncodingException {
String plain = URLDecoder.decode(encoded, StandardCharsets.UTF_8.toString());
LOGGER.info("Encoded text: {}, Plain text: {}", encoded, plain);
}
@Test
public void decodeTests() throws UnsupportedEncodingException {
decode("john%2Bdoe%40example.com");
decode("sample+text");
decode("%2B1653-124-23");
}
}
Code language: Java (java)
Run the above test in your favourite IDE and check the output. The encoded text is decoded using Java URL Decoder.
Output:
Code language: Shell Session (shell)Encoded text: john%2Bdoe%40example.com, Plain text: [email protected] Encoded text: sample+text, Plain text: sample text Encoded text: %2B1653-124-23, Plain text: +1653-124-23
Conclusion
Java provides utility classes URLEncoder and URLDecoder to encode/decode text using URLencoding to transfer data on http. In this tutorial we saw how to use Java URL encoder and decoder.
Download or browse the code on Github.
Github – source code
Reference
Further Read: Getting Started with Java 8 Lambda Expression