The basic idea this time is to present a way to form a tag cloud from user input text and text entered in the past. A tag cloud is a visual depiction of user-generated tags, or simply the word content of a site, used typically to describe the content of web sites. for this we will create an HTML form that will accept user text & also allow user to see tag cloud generated from mysql database which contains the text entered in the past.
This tag cloud was generated using the following data: tag_id;keyword;weight;link
“1”;”vimal”;”7″;”www.vimalkumarpatel.blogspot.com”
“2”;”scet”;”5″;”NA”
“3”;”engg”;”2″;”NA”
“4”;”0″;”1″;”NA”
“7”;”google”;”5″;”NA”
“8”;”cool”;”2″;”NA”
“9”;”orkut”;”3″;”NA”
<?php
echo '<form method="post" action="tag_cloud_gen.php" name="gen_tag_db">';
echo '<p>Input your text here:<textarea name="tag_input" rows="20" cols="80"></textarea></p>';
echo '<input type="submit" name="submit">';
echo '</form>';
?>
<h3>OR</h3>
<p>see the current tag cloud here</p>
<?php
echo '<form name="show_tag_cloud" method="post" action="show_tag_cloud.php">';
echo '<input type="submit" value="show current tag cloud" >';
echo '</form>';
?>
Code language: PHP (php)
The entered text will be tokenized into single words with php function strtok(), each of which will have its frequency counted and the pair will go into an array. This array will then be stored into a mysql database, we can optionally keep a coloumn in the mysql database table to store links if any for future expansion of this project. 1) tag_id —- int,primary key,auto increament 2) keyword — varchar(20),unique 3) weight — int 4) link — varchar(256). Next make a php file and name it tag_cloud_gen.php . The php code written in following lines just make an array ‘$words’ which has keyword in lower case & its frequency association from input text. The pairs from the array are then feed into the mysql database ‘tagcloud_db’ which has a table callet ‘tags’ whose columns are listed above. On encountering error the mysql _errrno() returns error number. While feeding the word array to the tags table duplication may occour, because of the previous entries, so we check whether this is the case by comparing the number returned to ‘1062’ which indicates duplicate field present in the table (the keyword coloumn of table has unique constraint). On encountering this we simply update the mysql database to include the count of this input word/tag too.<?php
///////////////////////////////////////////////////////////////////////////////////////////////////////
/**
* this function will update the mysql database table to reflect the new count of the keyword
* i.e. the sum of current count in the mysql database &amp;amp;amp; current count in the input.
*/
function update_database_entry($connection,$table,$keyword,$weight){
$string=$_POST['tag_input'];
$connection = mysql_connect("localhost", "root", "");
/**
* now comes the main part of generating the tag cloud
* we would use a css styling for deciding the size of the tag according to its weight,
* both of which would be fetched from mysql database.
*/
$query="select * from `tagcloud_db`.`tags` where keyword like '%$keyword%'";
$resultset=mysql_query($query,$connection);
if(!$resultset){
die('Invalid query: ' . mysql_error());
} else {
while($row=mysql_fetch_array($resultset)){
$query="UPDATE `tagcloud_db`.`tags` SET weight=".($row[2]+$weight)." where tag_id=".$row[0].";";
mysql_query($query,$connection);
}
}
}
?>
<?php
/*
* get the input string from the post and then tokenize it to get each word, save the words in an array
* in case the word is repeated add '1' to the existing words counter
*/
$count=0;
$tok = strtok($string, " \t,;.\'\"!&-`\n\r");//considering line-return,line-feed,white space,comma,ampersand,tab,etc... as word separator
if(strlen($tok)>0) $tok=strtolower($tok);
$words=array();
$words[$tok]=1;
while ($tok !== false) {
echo "Word=$tok";
$tok = strtok(" \t,;.\'\"!&-`\n\r");
if(strlen($tok)>0) {
$tok=strtolower($tok);
if($words[$tok]>=1){
$words[$tok]=$words[$tok] + 1;
} else {
$words[$tok]=1;
}
}
}
print_r($words);
echo '';
/**
* now enter the above array of word and corresponding count values into the database table
* in case the keyword already exist in the table then update the database table using the function 'update_database_entry(...)'
*/
$table="tagcloud_db";
mysql_select_db($table,$connection);
foreach($words as $keyword=>$weight){
$query="INSERT INTO `tagcloud_db`.`tags` (keyword,weight,link) values ('".$keyword."',".$weight.",'NA')";
if(!mysql_query($query,$connection)){
if(mysql_errno($connection)==1062){
update_database_entry($connection,$table,$keyword,$weight);
}
}
}
mysql_close($connection);
?>
Code language: PHP (php)
Make anether file and name it style.css . Put the following code in it.This will make our tag cloud look pretty, save it as style.css . again make a new php file and name it show_tag_cloud.php . In the php code that follows we connect to mysql database, fetch back all the tags, its weight and link. Then it calculates the size for each tag using its weight & minimum assumed size for tags, it also associates each tag the link retrieved from the database or with a google link if no link was there i.e. ‘NA’Code language: HTML, XML (xml)HTML, BODY { padding: 0; border: 0px none; font-family: Verdana; font-weight: none; } .tags_div { padding: 3px; border: 1px solid #A8A8C3; background-color: white; width: 500px; -moz-border-radius: 5px; } H1 { font-size: 16px; font-weight: none; } A:link { color: #676F9D; text-decoration: none; } A:hover { text-decoration: none; background-color: #4F5AA1; color: white; }
<?php
$connection = mysql_connect("localhost", "root", "");
$table="tagcloud_db";
$words=array();
$words_link=array();
mysql_select_db($table,$connection);
$query="SELECT keyword,weight,link FROM `tagcloud_db`.`tags`;";
if($resultset=mysql_query($query,$connection)){
while($row=mysql_fetch_row($resultset)){
$words[$row[0]]=$row[1];
$words_link[$row[0]]=$row[2];
}
}
// Incresing this number will make the words bigger; Decreasing will do reverse
$factor = 0.5;
// Smallest font size possible
$starting_font_size = 12;
// Tag Separator
$tag_separator = ' ';
$max_count = array_sum($words);
?>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<HTML>
<HEAD>
<TITLE> Tag Cloud Generator </TITLE>
<META NAME="Keywords" CONTENT="tag, cloud, php, mysql">
<META NAME="Description" CONTENT="A Tag Cloud using php and mysql">
<LINK REL="stylesheet" HREF="style.css" TYPE="text/css">
</HEAD>
<BODY>
<center><h1>Tag Cloud using php and mysql </h1><div align='center' class='tags_div'>
<?php
foreach($words as $tag => $weight )
{
$x = round(($weight * 100) / $max_count) * $factor;
$font_size = $starting_font_size + $x.'px';
if($words_link[$tag]=='NA') echo "<span style='font-size: ".$font_size."; color: #676F9D;'><a href='http://www.google.co.in/search?hl=en&q=".$tag."&meta='>".$tag."</a></span>".$tag_separator;
else echo "<span style='font-size: ".$font_size."; color: #676F9D;'><a href='http://".$words_link[$tag]."/'>".$tag."</a></span>".$tag_separator;
}
?>
</div></center>
</BODY>
</HTML>
Code language: PHP (php)
now put them all in your webserver’s root directory and watch the results. Each query will give you new results over time as the database grows. a sample output from my tag cloug looks like this: 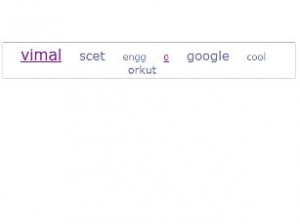
there is an errata in the second code,
please note that “<?” will not come at 9th line but between 30 & 31 line…
Hi Vimal,
Thanks for pointing out the typo in code. I have fixed it.
Can tou create a version that uses flash to do the same thing?
Hello,
Please add your site at http://www.sweebs.com. Sweebs.com is a place where other people can find you among the best sites on the internet!
Its just started and we are collecting the best found on the net! We will be delighted to have you in the sweebs listings.
Regards
Kris
When is tags puts in quote? eg
“a href=’thread.php?increment=-1′>></ ““
@The Deer
well a flash enabled tag cloud is quiet a flashy idea for the time being,
why i think that is because of following two reasons
1. It makes page heavier, it terms if size and time to load.
2. It will not provide links to a web crawler from search engines like google, msn or yahoo, as none of these search engines have the capability to search for text inside a flash or image file.
over that i am not much familiar with flash :(
but i liked the idea and once enough information about flash is gathered i may try it out :)
I already try this code, but I get error :
Warning: mysql_select_db(): supplied argument is not a valid MySQL-Link resource in D:\xampp\htdocs\tags\tag_cloud_gen.php on line 59
Is it problem with the connecttion?
am getting errors with this code…PLEASE HELP
Array ( [0] => 1 )
Warning: mysql_select_db(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 59
Warning: mysql_query(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 62
Warning: mysql_errno(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 63
Warning: mysql_close(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 68
ALSO, ONE OF THE USER MENTIONED AN ISSUE ON LINE 9 OF SECOND FILE, BUT I DIDN’;T GET ANY ISSUE HERE…PLEASE SUGGETS
Hi Prazetyo / Madhuprasad,
I think there was some typo error in the code. I have updated the code and removed the error. Please take the latest code from above article and try again. It should work :)
When I submit keywords, this all I get from the \"tag_cloud_gen.php\" page:
Array ( [0] => 1 )
And it\’s just inserting a \"0\" into the database for the string.
Please help.
thanks,
MD
Hi, does anyone know of example code somewhere that does not make use of a database, but merely makes a tag cloud from text entered by the user into a form?
I\’m having the same problem as Madhur Prasad. Is there any fix for this?
I\’m getting the same error Array ( [0] => 1 )
Where can I download this tutorial?
Hey..
thanks a ton for the post..
came in really handing.. n it works without a flaw..
ive implemented it without understandin the logic entirely..
time to go thru wat uve written.
thanks anyway.. \m/
Hi, thank you very much …
Im trying to figure out how to create a cloud tag for an html web site any help would be appreciated. Thanks
I’m having the same problem:
“Array ( [0] => 1 )
Warning: mysql_select_db(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 59
Warning: mysql_query(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 62
Warning: mysql_errno(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 63
Warning: mysql_close(): supplied argument is not a valid MySQL-Link resource in c:\wamp\www\final\tag\tag_cloud_gen.php on line 68”
Shame you aren’t replying to the Array ( [0] => 1 errors above. I have commented and uncommented every line of the script trying to debug. No luck. I have checked database connections and debugged them as well
I am sure you realize that you reference $table as a database and not a table in the last few lines of your code, but that isn’t the problem either.
I would love to see this working.
Can someone help me? I am getting this ERROR Array ( [0] => 1 )
Thanks for this , its really helpful!
I’m not even a great programmer and figured it out. Wow.
Added:
$string=$_POST[‘tag_input’];
after function in tag_cloud_gen.php
posted value from for was not being set to $string
My only issue now is if the tags exist, its adding them again and not just updating the keyword(s) weight.
Generating Tag cloud with PHP and MySQL post for code thanx.
Great one.. Just took me 10 minutes to implement this into my website and looks awesome now..
Hmm, my tags aren’t wrapping . . . http://www.kate-maher.com/kiva649/tag_cloud.php, otherwise, great example! thanks!
I just put the followings lines out of the function and the code works!
$string=$_POST[‘tag_input’];
$connection = mysql_connect(“localhost”, “root”, “”);
great example! thanks!
Works well. Had a few problems with the MySQL queries, but they were easy to fix.
I changed the code around to use GET instead of POST, for two reasons.
1. They can do a new search from the url directly
2. They are able to refresh the page without getting the submit data popup.
Then I just record by IP so they don’t record a search within a 10 minute time frame.
All in all, good code Vimal. Thanks.
This is one of the best tutorial i found in the internet. He explained in a very good manner. The layman can understand with this kind of explanation. Real great work viral patel..keep going..
Could you please help, how did u get it working. I’m still getting this error: Array ( [0] => 1 )
I’m still getting Array ( [0] => 1 ) in tag_cloud_gen.php. Any help would be appreciated.
Array ( [0] => 1 )
Warning: mysql_select_db(): supplied argument is not a valid MySQL-Link resource in E:\php\xampp\htdocs\ranjan\extra\test\tag_cloud_gen.php on line 69
Warning: mysql_query(): supplied argument is not a valid MySQL-Link resource in E:\php\xampp\htdocs\ranjan\extra\test\tag_cloud_gen.php on line 72
Warning: mysql_errno(): supplied argument is not a valid MySQL-Link resource in E:\php\xampp\htdocs\ranjan\extra\test\tag_cloud_gen.php on line 73
Warning: mysql_close(): supplied argument is not a valid MySQL-Link resource in E:\php\xampp\htdocs\ranjan\extra\test\tag_cloud_gen.php on line 78
I am getting These Error. Any one can hepl me to sort out this problem
tanx a lot dear Vimal Patel
Sir,Can Give me code for the web chat using php and mysql ……….
why this code have a more than 100 ERROR :-)
THIS CODE NOT TESTED AND ONLY FOR THE TUTORIALS !!!!
Hi There !
Thanks for such an desired codes, I was just waiting to complete this in my admin and now it seems to be completed – all thanks to this post.
I want to know that is there a way to stop error reporting in WAMP where as the same error is not shown when tried on online server. Also, this error comes only when we try to save more than 1 tags using comma or space or separator targeting a Loop section i.e.
/*
* get the input string from the post and then tokenize it to get each word, save the words in an array
* in case the word is repeated add ‘1’ to the existing words counter
*/
$tok = strtok($string, ” t,;.'”!&-`nr”);//considering line-return,line-feed,white space,comma,ampersand,tab,etc… as word separator
if(strlen($tok)>0) $tok=strtolower($tok);
$words=array();
$words[$tok]=1;
while ($tok !== false) {
echo “Word=$tok”;
$tok = strtok(” t,;.'”!&-`nr”);
if(strlen($tok)>0) {
$tok=strtolower($tok);
if($words[$tok] >= 1){
$words[$tok]=$words[$tok] + 1;
} else {
$words[$tok]=1;
}
}
}
print_r($words);
echo ”;
The Error begins from the – if($words[$tok] >= 1){
For reference I have a uploaded a image – http://rhs.r4y.in/images/tag-cloud-error.jpg
Thanks in Advance…
Please provide me solution if there is any…
I have used this script recently and it works fine, also, it is working on online server also, but there is a small issue that is when I run this script on localhost everything works fine and all data is stored as desired but there shows an error after execution on browser on localhost whereas same codes same db same all stuff works fine on online server. How to hide those error while creating programs offline on localhost and in testing mode of a project.
Help appreciable and codes highly appreciated…